Uncovering Tarot Biases with Simple NLP
By Artyom Bologov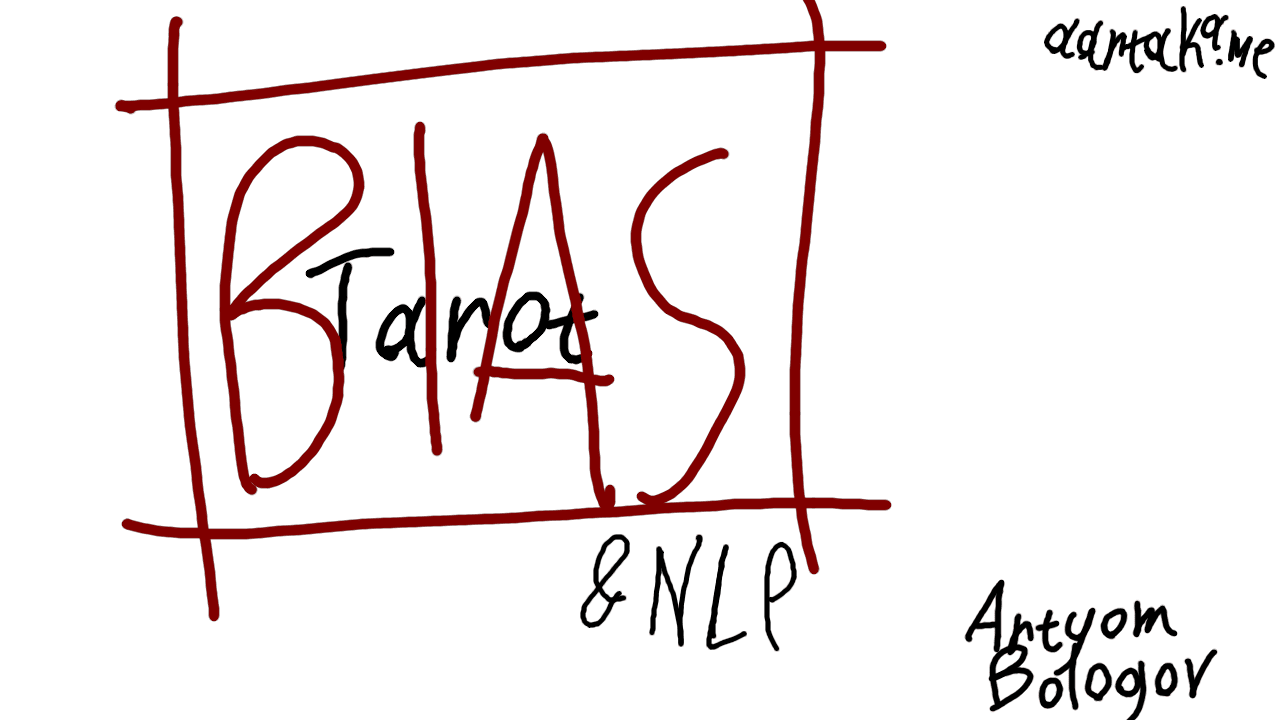
I picked up a really good Tarot pack. Some Russian Orthodox sect one. With bright solid colors, all unlike typical faded hues of other Rider-Waite decks. And with a "Guide to divination from the Holy Spirit." Sham, of course, but a funny one.
I turned to other sources for reliable (ha!) interpretations. One of my sources is learntarot.com. It's vintage, brutalist, and personal. Another source is The Illustrated Key to the Tarot by L. W. De Laurence. Mostly just a reskin of original Waite's book, but a representative one nonetheless. It's listing much more controversial and counter-intuitive interpretations. So I like it. Except for the "dark man" and "military" parts, of course.
Based on these, I hacked together my own Tarot page. Mainly for me to consult with when on the phone. It uses De Lawrence's interpretations, because they are more fun and varied.
Doing all this reading and indexing I can't help but notice a trend: There are more "male" cards than "female" ones. There are more White European archetypes than persons of color. Most card interpretations are disgustingly positive. But is that just my impression or there's structural problem with Tarot? In this post, I'm applying (extremely simple) NLP (Natural Language Processing) methods to learn the sexist and racist secrets of Tarot
Preliminaries #
I used my Tarot 3.2 page to get card description.
And I dumped these to a file of the (essentially TSV) format
id[tab]name[tab]upright-meaning[tab]reversed-meaning[tab]card-description
Such format is easy to reproduce and parse in any language.
Including (my lovely) Lisp:
(defparameter file "~/junkyard/cards.txt")
(defun parse-cards (file)
(mapcar (lambda (line)
(uiop:split-string line :separator '(#Tab)))
(uiop:read-file-lines file)))
(defparameter cards (parse-cards file))
;; Shared between most of code pieces below.
(defun words (string)
(remove-if 'uiop:emptyp
(uiop:split-string string :separator " ,.!;:?")))
With data nicely preprocessed, we have to uncover some biases.
Overview: Word Frequencies #
Another NLP thing I'm doing is looking at word frequences.
With these, I can see what cards and their interpretations are about.
And what other issues there might be.
(defparameter words
(reduce 'append
(mapcar 'words
(mapcar 'string-downcase (mapcar 'third cards)))))
(defun most-frequent-words (words)
(let ((freqs (make-hash-table :test 'equal)))
(dolist (word words)
(uiop:ensure-gethash word freqs (constantly 0))
(incf (gethash word freqs)))
freqs))
(defparameter sorted-frequent
(loop for word being the hash-key of (most-frequent-words words)
using (hash-value frequency)
collect (cons word frequency)
into interim
finally (return (sort interim '> :key 'cdr))))
And now to get actual most frequent words:
(subseq sorted-frequent 0 150)
;; => (("the" . 119) ("a" . 100) ("of" . 95) ("and" . 53) ("is" . 50) ("in" . 45) ("card" . 32) ("also" . 30) ("it" . 30) ("for" . 28)
;; ("man" . 20) ("to" . 17) ("that" . 17) ("as" . 16) ("which" . 16) ("but" . 16) ("if" . 15) ("on" . 15) ("querent" . 14)
;; ("success" . 13) ("may" . 12) ("or" . 12) ("be" . 12) ("are" . 12) ("with" . 12) ("good" . 12) ("will" . 11) ("woman" . 11)
;; ("by" . 11) ("young" . 11) ("marriage" . 10) ("another" . 10) ("these" . 10) ("dark" . 10) ("love" . 9) ("an" . 9) ("his" . 9)
;; ("business" . 9) ("who" . 8) ("has" . 8) ("fortune" . 8) ("this" . 8) ("design" . 8) ("one" . 8) ("from" . 8) ("loss" . 7)
;; ("great" . 7) ("not" . 7) ("generally" . 7) ("all" . 7) ("signifies" . 7) ("its" . 7) ("news" . 7) ("person" . 6) ("other" . 6)
;; ("favorable" . 6) ("intelligence" . 6) ("he" . 6) ("some" . 6) ("law" . 5) ("reading" . 5) ("readings" . 5) ("lady" . 5) ("bad" . 5)
;; ("family" . 5) ("things" . 5) ("fair" . 5) ("account" . 4) ("triumph" . 4) ("felicity" . 4) ("contentment" . 4) ("position" . 4)
;; ("concord" . 4) ("gifts" . 4) ("been" . 4) ("sadness" . 4) ("there" . 4) ("cards" . 4) ("money" . 4) ("unexpected" . 4) ("skill" . 3)
;; ("enemies" . 3) ("male" . 3) ("wisdom" . 3) ("science" . 3) ("power" . 3) ("reason" . 3) ("authority" . 3) ("whom" . 3) ("war" . 3)
;; ("trouble" . 3) ("destruction" . 3) ("force" . 3) ("deception" . 3) ("happiness" . 3) ("change" . 3) ("flight" . 3) ("well" . 3)
;; ("crown" . 3) ("usually" . 3) ("prosperity" . 3) ("friendship" . 3) ("state" . 3) ("gives" . 3) ("suggestion" . 3) ("search" . 3)
;; ("help" . 3) ("absence" . 3) ("her" . 3) ("vigilance" . 3) ("repose" . 3) ("have" . 3) ("hope" . 3) ("girl" . 3) ("life" . 3)
;; ("death" . 3) ("service" . 3) ("sense" . 3) ("so" . 3) ("enterprise" . 3) ("inheritance" . 3) ("riches" . 3) ("trade" . 3)
;; ("towards" . 3) ("gain" . 3) ("several" . 3) ("surface" . 3) ("friend" . 3) ("him" . 3) ("child" . 3) ("honor" . 3) ("friendly" . 3)
;; ("pleasure" . 3) ("matter" . 3) ("perfection" . 3) ("happy" . 3) ("gift" . 3) ("new" . 3) ("reflection" . 3) ("address" . 2)
;; ("sickness" . 2) ("pain" . 2) ("secrets" . 2) ("mystery" . 2) ("future" . 2) ("interests" . 2) ("female" . 2) ("action" . 2)
;; ("protection" . 2) ("trials" . 2))
Some highlights:
- the, a, of, and, it, but, are etc.
- Swadesh list, expectable.
- (man 20) (woman 11)
- Okay, so there's a gender disbalance? Need to check some more.
- good, love, great, favorable, fair, triumph, felicity, contentment
- These are too frequent. We need to do something about it. And we'll do—later.
- loss, bad, sadness, enemies, war, trouble, destruction
- Notice that these appear near the end of the list. They are frequent and diverse, but not as frequent as "good" terms. Or are they?
- dark
- Notice that it's not the same thing as above. "Dark" is used by De Laurence in phrases like "dark man" or "dark woman," and not in the emotionally darker sense. Which is cool, because it introduces more diversity into cards, but is that enough? Have to check.
Word frequencies are boring, but they revealed three directions for future research! Let's tackle them:
Gender Disbalance: Wordlist Matches #
So my most pronounced complaint about Tarot is the gender balance. Or the absence of such balance, rather. Most cards feature males, and males only. Major Arcana is somewhat gender-balanced, but that's about it. So how about measuring the dire state of things?
I'm going to use a simple list of "male" and "female" indicator words.
And then finding these in the card description.
Luckily, the descriptions are pretty simplistic and parseable in De Lawrence's book.
(defparameter male-indicators
'("man" "male" "masculine" "he" "his" "him" "knight" "king" "husband"))
(defparameter female-indicators
'("woman" "female" "feminine" "she" "her" "queen" "maiden" "wife"))
(defparameter male-cards 0)
(defparameter female-cards 0)
(defparameter collective-cards 0)
(defparameter neutral-cards 0)
(dolist (card cards)
(destructuring-bind (id _ _ _ description)
card
(let ((has-male (intersection (words description) male-indicators
:test 'string-equal))
(has-female (intersection (words description) female-indicators
:test 'string-equal)))
(cond
((and has-male has-female)
(incf collective-cards))
(has-female
(incf female-cards))
(has-male
(incf male-cards))
(t
(incf neutral-cards))))))
;; Pages are often interpreted as female, thus +4 Pages
(incf female-cards 4)
I've introduced the "collective" and "neutral" categories.
For cards that feature both males and females.
And for cards where none are present (usually aces or cards featuring children.)
(list male-cards female-cards collective-cards neutral-cards)
;; => (38 17 7 20)
Not cool. "Male" cards are more than twice as prevalent as "female" ones! Even "neutral" cards are more prevalent than "female" cards! Even adding "collective" cards doesn't tip the scales significantly! So yes, Tarot is unnaturally male-centric.
Suits and Races: Manual Accounting #
De Lawrence lists mapping from suits to person appearance:
- Wands
- "very fair people, with yellow or auburn hair, fair complexion and blue eyes"
- Cups
- "people with light brown or dull fair hair and grey or blue eyes"
- Swords
- "people having hazel or grey eyes, dark brown hair and dull complexion"
- Pentacles
- "very dark brown or black hair, dark eyes and sallow or swarthy complexions"
White people (arguably) occupy three out of four suits. Pentacles can (barely) be interpreted as persons of color. Some other cards also mention "dark men/women", so let's count them. Manually, unfortunately:
- Seven of Swords
- Seven of Wands
- Page of Wands
- Knight of Wands
- Queen of Wands
- King of Wands
- Eight of Pentacles
So we have all (14) Pentacles. Then—surprisingly—four court cards from Wands! And some smaller cards from other suits. In total, "dark" persons of color occupy 21 card out of 56 Lesser Arcana cards. Which is by no means congruent with world population. But it's something.
Positivity: Sentiment Analysis #
Now to the most fun (literally) part of the post. Assessing the positivity bias of Tarot.
There's a perfect tool for that mood checking—Sentiment Analysis.
Basically going through "positive" and "negative" words.
And seeing which are present in the text.
I might've done it with wordlists.
But the wordlists would grow unbounded and take a ton of time to compose.
So I'm using an existing Lisp library,
cl-sentiment.
(ql:quickload :cl-sentiment)
(cl-sentiment:initialize)
(defparameter upright-sentiments
(mapcar (lambda (card)
(destructuring-bind (id _ upright reversed _)
card
(list id (cl-sentiment:sentiment upright))))
cards))
(defparameter reversed-sentiments
(mapcar (lambda (card)
(destructuring-bind (id _ upright reversed _)
card
(list id (if (uiop:emptyp reversed)
0
(cl-sentiment:sentiment reversed)))))
cards))
(defun average (list)
(/ (reduce '+ list) 78))
(average (mapcar 'second upright-sentiments))
;; => 133/78 (1.7051282, 6650/39%)
(average (mapcar 'second reversed-sentiments))
;; => -47/26 (-1.8076923, -2350/13%)
A surprising result. Yes, positive cards are extremely prevalent in upright positions. But! Reversed cards are balancing this out perfectly. So I guess I have to use reversed cards now.
Further Directions #
Some things that I was either too lazy or too stupid to do:
- Vector embeddings and their comparisons.
- Description clustering.
- Neural networks and free prompting for biases.
Conclusion: Biases #
To summarize the list of faults Rider-Waite-derived decks have:
- "Male" card prevalence, more than two times the count of "female" cards.
- Racial misrepresentation.
- And overly positive upright cards, unless you're using reversed cards too.
It's not much, true. But it still makes most spreads be about rich happy white men. Not the most representative archetype. We're past that. Let Tarot be past that too.
Thanks for reading! Go make a spread for yourself with something like Modern Witch Tarot or Fyodor Pavlov's beautiful deck and enjoy the day that's totally not influenced by Tarot spreads and biases!